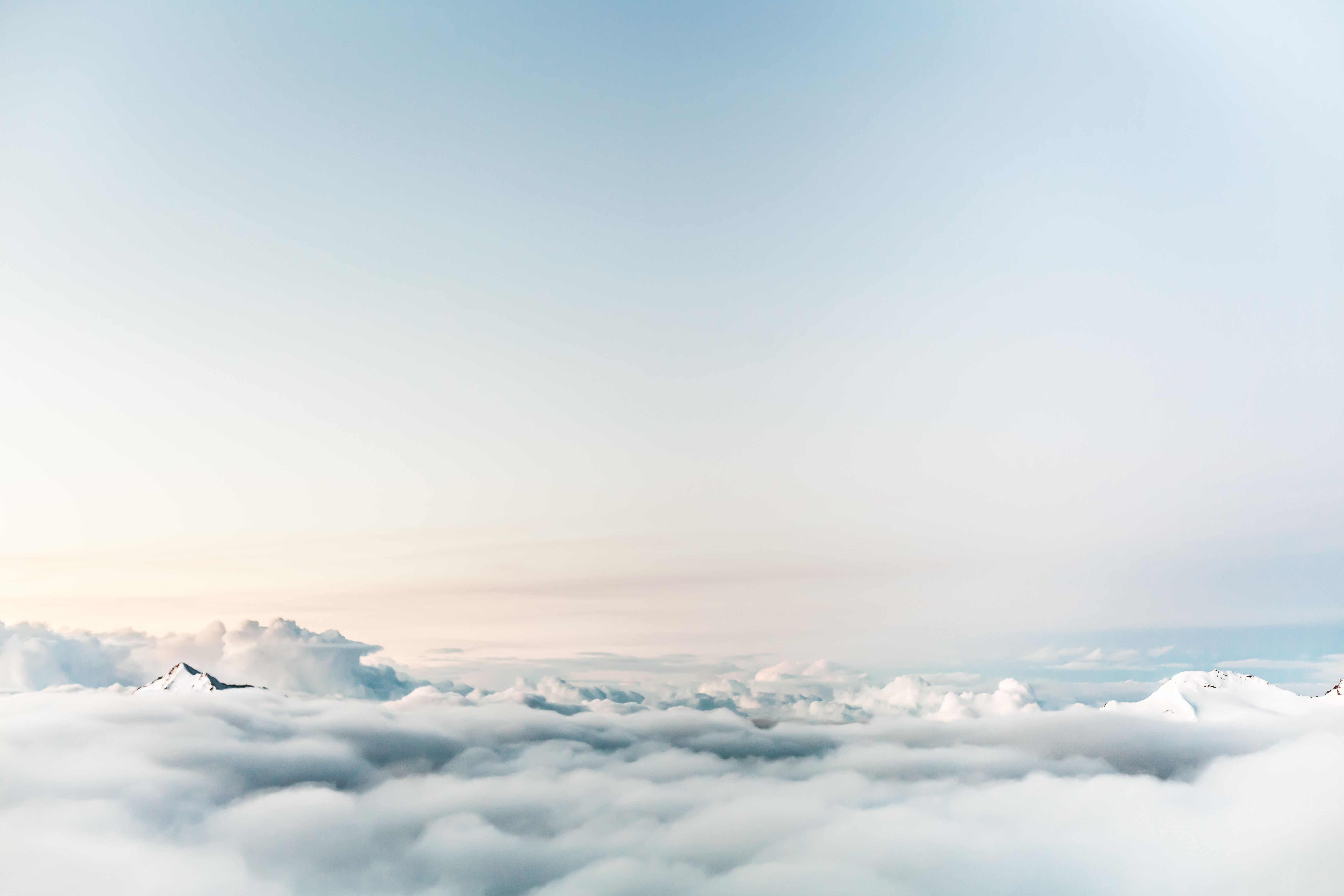
Deploy your Java Web App to Heroku
Table of Contents
Requirements and Preparation
Unfortunately Heroku currently only Supports Tomcat version 9.x. As a consequence you're not able to use the Java Jakarta Servlets, since they are only supported with Tomcat version 10.x.
As a consequence you need to replace all your Jakarta Servlet imports with the "old-school" Javax Serverlets:
find . -type f -name "*.java" -print0 | xargs -0 sed -i '' -e 's/import jakarta/import javax/g'
Delete those dependencies from your pom.xml:
<dependency>
<groupId>jakarta.servlet</groupId>
<artifactId>jakarta.servlet-api</artifactId>
<version>${servlet.version}</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>jakarta.servlet.jsp.jstl</artifactId>
<version>${jstl.version}</version>
</dependency>
Add those dependencies to your pom.xml:
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
</dependency>
<dependency>
<groupId>jstl</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
we also need the Heroku web-runner packet in the pom.xml:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>3.3.0</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>copy</goal>
</goals>
<configuration>
<artifactItems>
<artifactItem>
<groupId>com.heroku</groupId>
<artifactId>webapp-runner</artifactId>
<version>9.0.69.1</version>
<destFileName>webapp-runner.jar</destFileName>
</artifactItem>
</artifactItems>
</configuration>
</execution>
</executions>
</plugin>
let's also change the Jakarta xml header on the web.xml file from:
<web-app version="5.0" xmlns="https://jakarta.ee/xml/ns/jakartaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/jakartaee https://jakarta.ee/xml/ns/jakartaee/web-app_5_0.xsd">
to:
<web-app version="4.0" xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd">
Build the App
Browse to the root folder of your Java Application:mvn clean package
next run:java -jar target/dependency/webapp-runner.jar target/*.war
That’s it. Your application should start up on port 8080. If this works as expected, your ready to deploy your App to Heroku.
Deploy to Heroku
Create a Procfile in the root folder of your Application:echo 'web: java $JAVA_OPTS -jar target/dependency/webapp-runner.jar --port $PORT target/*.war' > Procfile
We also need to declare the Java version to Heroku:echo 'java.runtime.version=17' > system.properties
Commit your changes to Git:git init
git add .
git commit -m "Ready to deploy"
Create the app and deploy your code:heroku create
--region eu
git push heroku main
Your web app should now be up and running on Heroku. Open it in your browser with:heroku open