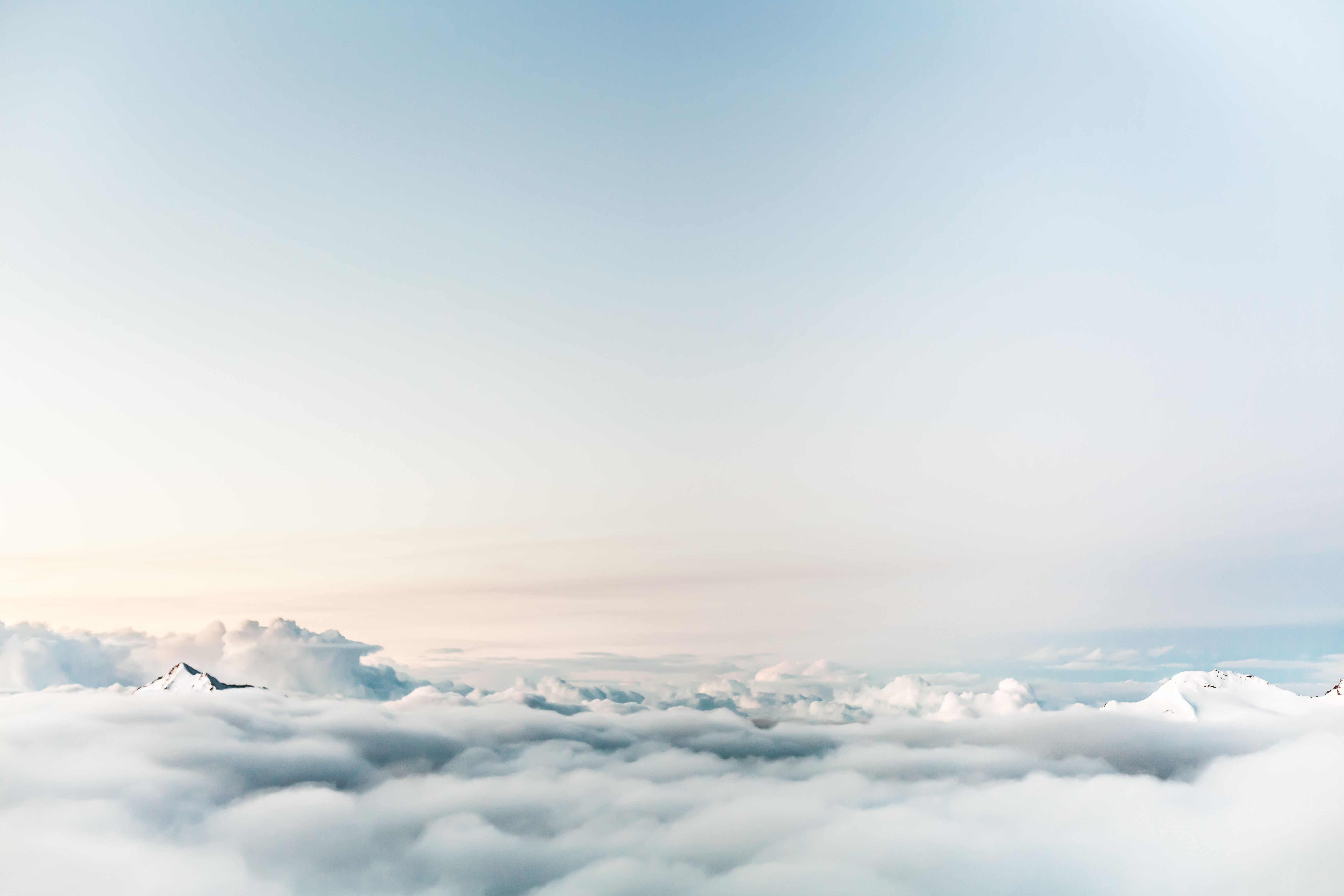
JavaScript arrays
Table of Contents
Array
- An array combines multiple values in a single variable
- Arrays are helpful if the number of values is huge or dynamic
- Each value of an array has a unique numerical index
// Normal variables hold exactly one value
const colorA = "blue";
const colorB = "red";
const colorC = "yellow";
// An array variable can hold more than one value
const colors = ["blue", "red", "yellow"];
// Each value of the array can be accessed by a numerical index
console.log(colors[0]); // >> blue
console.log(colors[1]); // >> red
console.log(colors[2]); // >> yellow
Creating an Array
- An array can be created as a literal or by using the array constructor (not recommended)
- The values of an array may have different types
// Array literal (recommended)
const a = ["one", "two", "three", "four"];
// Array constructor (not recommended)
const b = new Array("Apple", "Mango", "Kiwi");
const c = new Array(3, 4); // creates [3, 4]
const d = new Array(3); // creates [, , ] (three empty solots)
// Array with different types
const e = ["Apple", 5, true, 4.25, null];
Modifying an Array
- Elements can be modified and inserted using the index operator
- In addition, there are many useful methods to work with arrays (see developer.mozilla.org)
const a = [7, 8, 40];
// Change value
a[2] = 45; // [7, 8, 45]
// Insert new values
a[a.length] = 17; // [7, 8, 45, 17]
a[6] = 23; // [7, 8, 45, 17, , , 23] (length: 7)
console.log(a[5]); // >> undefined
// Remove some elements
a.splice(2, 4); // [7, 8, 23]
// Use array as stack
a.push(12); // [7, 8, 23, 12]
console.log(a.pop()); // >> 12 (a: [7, 8, 23])
FIFO and LIFO
No these are not Dogs. It's about two different concepts and the main difference between a Queue and a Stack:
- FIFO = first in first out -> that's a queue
- LIFO = last in first out -> that's a stack
In JavaScript we use push
to put's it on top of stack and pop
to pull an element from the top of a stack
Loop Through an Array
There are different ways to iterate over the elements of an array:
const colors = ["blue", "red", , "black"];
// Using for loop
for (let i = 0; i < a.length; i++) { // >> 0 -> blue
console.log(i+" -> "+a[i]); // >> 1 -> red
} // >> 2 -> undefined
// >> 3 -> black
// Using for...of loop
for (let color of colors) { // >> blue
console.log(color); // >> red
} // >> undefined
// >> black
// Using forEach method (empty slots are skipped)
a.forEach(function(item, index) { // >> 0 -> blue
console.log(index+" -> "+item); // >> 1 -> red
}); // >> 3 -> black
Arrays are Objects
- Arrays in JavaScript are based on numerical indexes
- Named indexes access properties of an object
- Since arrays are objects, both types of indexes can be used but address different concepts
const colors = ["blue", "red", "black"];
console.log(colors.length); // >> 3
// Named indexes address object properties and not array values
colors["favorite"] = "pink";
console.log(colors.favorite); // >> pink
console.log(colors.length); // >> 3 (still!)
colors.forEach(function(item, index) { // >> 0 -> blue
console.log(index+" -> "+item); // >> 1 -> red
}); // >> 2 -> black
for (let i in colors) { // >> 0 -> blue
console.log(i+" -> "+colors[i]); // >> 1 -> red
} // >> 2 -> black
// >> favorite -> pink